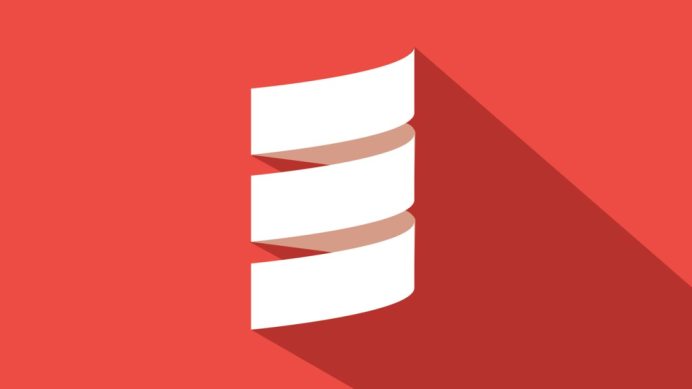
What are implicit classes?
Implicit classes are a feature that allow you to extend the functionality of existing types by adding new methods. They are defined using the implicit
keyword and have a single constructor parameter. We can use these class as if they belong to the original type without having to perform explicit type conversion.
Implicit classes are particularly useful for adding utility methods to existing types. They allow you to do this without creating a new type or modifying the original type. You can also use implicit classes to add implicit conversions. It can be helpful in making your code more concise and readable.
Should implicit classes always extend AnyVal in scala?
Implicit classes in Scala do not have to extend AnyVal
. They can extend any type that is a subtype of Any
. However, if the implicit class is meant to be used as a value type and is simple enough, it may make sense to extend AnyVal
to allow for optimized storage and improved performance.
It’s worth noting that an implicit class that extends AnyVal
can only have a single constructor parameter and is subject to certain restrictions in terms of its functionality, as it is meant to represent a value type. On the other hand, implicit classes that do not extend AnyVal
are treated as normal classes and can have multiple constructor parameters, additional fields, and more complex logic.
So whether or not an implicit class should extend AnyVal
depends on the specific use case and the intended behavior of the class.
Examples:
Here’s an example to illustrate the difference between implicit classes that extend AnyVal
and those that do not.
Let’s say we want to add a method to the Int
type that squares its value. We can define an implicit class that takes an Int
value and adds this method:
implicit class IntOps(val x: Int) extends AnyVal {
def square: Int = x * x
}
In this case, the implicit class extends AnyVal
, so it is optimized for use as a value type. We can use this implicit class like this:
scala> 5.square
res0: Int = 25
Now, let’s say we want to add a similar method to the String
type that repeats its value a specified number of times. To do this, we can define an implicit class that takes a String
value and adds this method:
implicit class StringOps(val s: String) {
def repeat(n: Int): String = s * n
}
In this case, the implicit class does not extend AnyVal
, because it is not meant to be used as a value type. We can treat it as a normal class. We can use this implicit class like this:
scala> "Hello ".repeat(3)
res1: String = Hello Hello Hello
So, in this example, the implicit class that extends AnyVal
is more optimized for performance as a value type, while the implicit class that does not extend AnyVal
is treated as a normal class and can handle more complex logic.
Here’s one more example. Let’s say we want to add a method to the Int
type that calculates the factorial of a number. We can define an implicit class that takes an Int
value and adds this method:
implicit class IntFactorial(val n: Int) {
def factorial: Int = {
def fact(x: Int, acc: Int): Int =
if (x <= 1) acc else fact(x - 1, acc * x)
fact(n, 1)
}
}
In this case, the implicit class does not extend AnyVal
, because it needs to perform a recursive calculation, which is not possible with value types. We can use this implicit class like this:
scala> 5.factorial
res2: Int = 120
So, in this example, we see that extending AnyVal
is not always the right choice, as the more complex logic required by the factorial
method makes it more appropriate to use a normal class that does not extend AnyVal
.
Conclusion:
In conclusion, whether or not an implicit class in Scala should extend AnyVal
depends on the intended use case and behavior of the class. If the implicit class is meant to be used as a simple value type, then extending AnyVal
can result in improved performance and optimized storage. On the other hand, if the implicit class requires more complex logic or additional fields, it may make more sense to treat it as a normal class and not extend AnyVal
. In either case, implicit classes can be a convenient way to add new methods to existing types in Scala, and the choice of whether to extend AnyVal
or not should be based on the specific requirements of each case.
